Debugbar for Laravel
利用 laravel-debugbar 套件除錯
利用composer安裝
由於只有在開發時才需要,所以加入–dev參數
composer require barryvdh/laravel-debugbar --dev
安裝過程的劃面如下
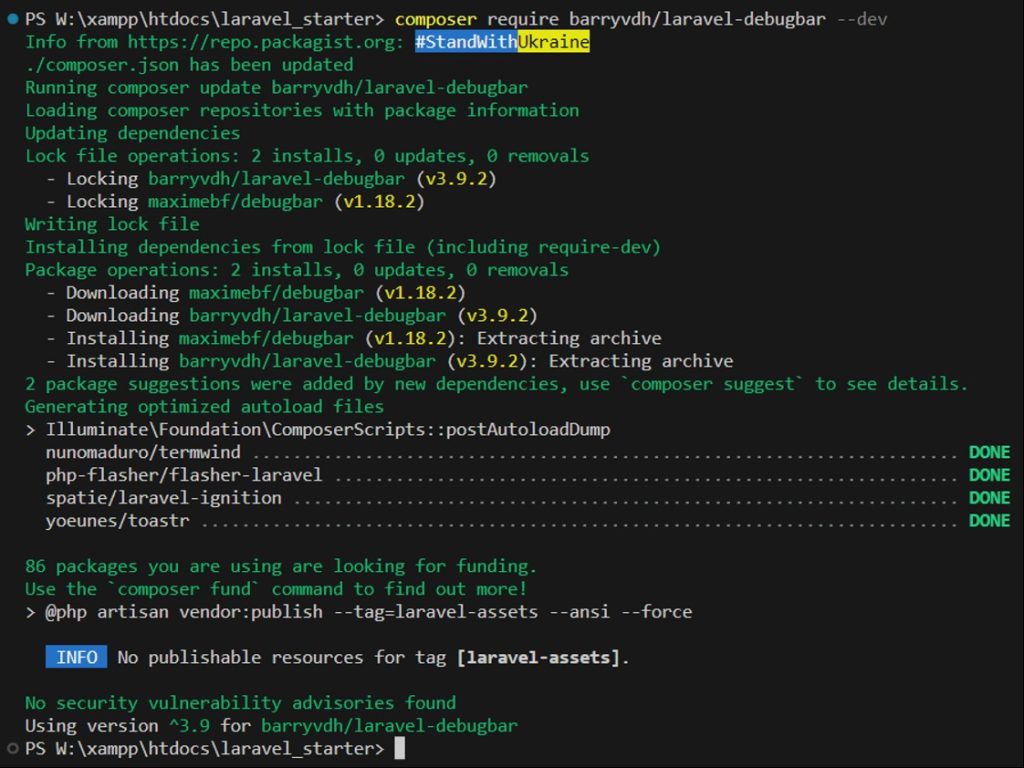
安裝完後, composer.json 會在 require-dev 加入 “barryvdh/laravel-debugbar”
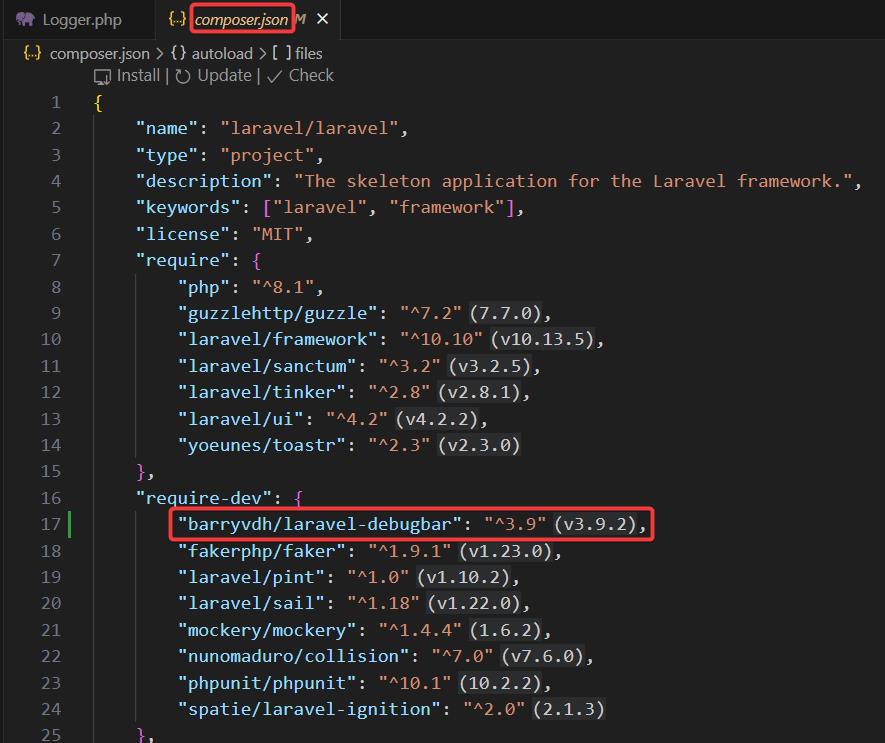
Debugbar 不需手動加入 ServiceProvider , 只要在 config\app.php 中的 APP_DEBUG 設為 true,它就會自動偵測起動。The Debugbar will be enabled when APP_DEBUG is true.在流覽器中就可以看到底部有 Laravel Debugbar。
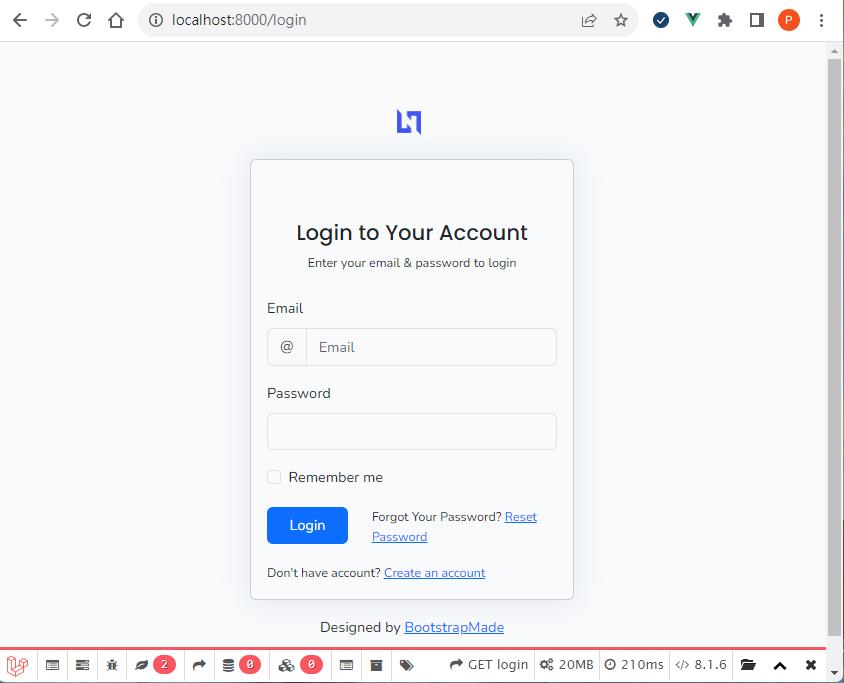
不要自動偵測 Laravel without auto-discovery
If you don’t use auto-discovery, add the ServiceProvider to the providers array in config/app.php
'providers' => [
....
Barryvdh\Debugbar\ServiceProvider::class,
],
'aliases' => [
....
'Debugbar' => Barryvdh\Debugbar\Facade::class,
]
執行以下語法以複製相關檔案 Copy the package config to your local config with the publish command:
php artisan vendor:publish --provider="Barryvdh\Debugbar\ServiceProvider"
使用方式
在程式段裡的運用
use Barryvdh\Debugbar\Facades\Debugbar;
class HomeController extends Controller
{
/**
* 有認證則導向 Dashboard 頁面, 否則導向 login 頁面
*/
public function dashboard()
{
// 檢查是否已有認證成功
if (Auth::check()) {
Debugbar::debug(Auth::user()); //查詢登入者資訊
return view('dashboard');
}
toastr()->error('Oops! You have sign in first!', 'Oops!');
return redirect()->route('login')
->with('message', 'Opps! You are not allowed to access')
->withErrors([
'email' => 'Your provided credentials do not match in our records.',
])->onlyInput('email');
}
}
帳號登入後,讀取帳號資訊
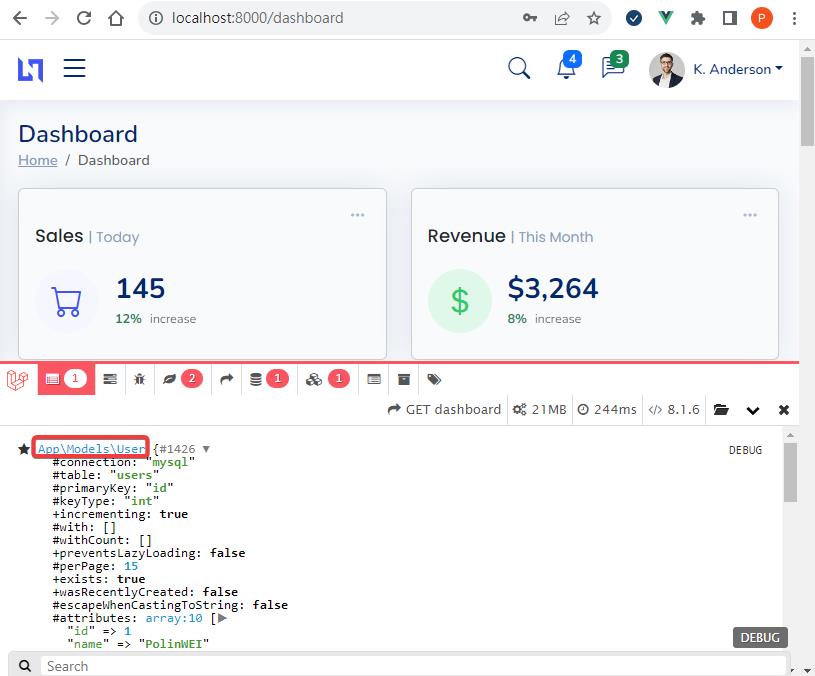
其它使用方法
// 可以在程式段加入下列程式碼
Debugbar::info($object);
Debugbar::error('Error!');
Debugbar::warning('Watch out…');
Debugbar::addMessage('Another message', 'mylabel');
// And start/stop timing:
Debugbar::startMeasure('render','Time for rendering');
Debugbar::stopMeasure('render');
Debugbar::addMeasure('now', LARAVEL_START, microtime(true));
Debugbar::measure('My long operation', function() {
// Do something…
});
// 或者 log exceptions:
try {
throw new Exception('foobar');
} catch (Exception $e) {
Debugbar::addThrowable($e);
}
There are also helper functions available for the most common calls:
// All arguments will be dumped as a debug message
debug($var1, $someString, $intValue, $object);
// `$collection->debug()` will return the collection and dump it as a debug message. Like `$collection->dump()`
collect([$var1, $someString])->debug();
start_measure('render','Time for rendering');
stop_measure('render');
add_measure('now', LARAVEL_START, microtime(true));
measure('My long operation', function() {
// Do something…
});
發佈留言